In this post we will see File operation offered by Python. We will see in detail how to operate and manipulate File using Python along with some real time examples.
Python comes with inbuilt library which is not required to import them to perform the operations. Lets get started,
Opening an Existing File
IN[1]
def main():
f = open('example.txt', 'r')
if __name__ == "__main__" :
main()
OUT[1]
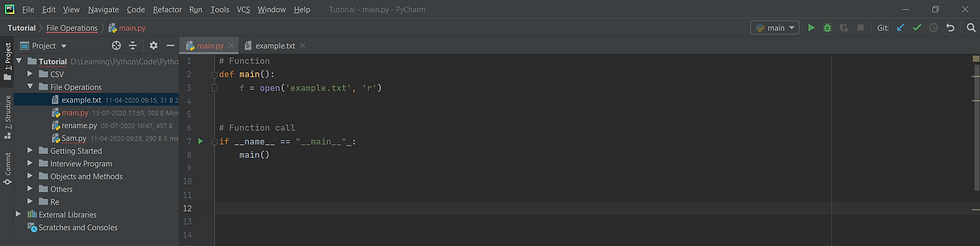
EXPLANATION
By default, python has an in-build function named open(), which accepts file name and file type as arguments. This provide the object created to have access to the file based on the file name. Below there are the other file type on which we can open a file,
"r" : Read , Default value. Opens a file for reading, error if the file does not exist
"a" : Append , Opens a file for appending, creates the file if it does not exist
"w" : Write , Opens a file for writing, creates the file if it does not exist
"x" : Create , Creates the specified file, returns an error if the file exists
"t" : Text , Default value. Text mode
"b" : , Binary , Binary mode (e.g. images)
Creating a New File
IN[2]
def main():
f = open('example1.txt', 'x')
if __name__ == "__main__" :
main()
OUT[2]
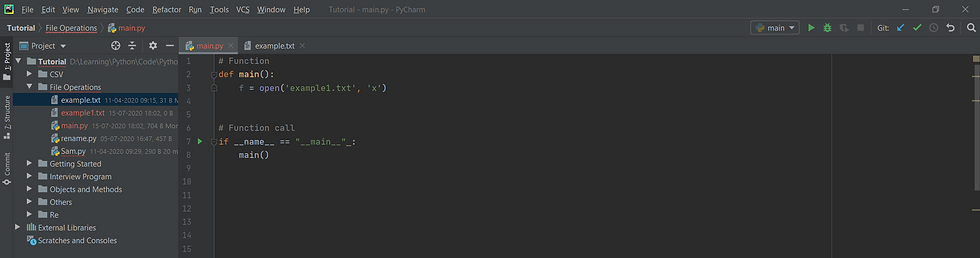
EXPLANATION
From our first snapshot you can see the right side panel that we have only one txt file named example and after executing above code we have to txt file, this because we used open() which file type x. This will create a new file which was passed as an argument.
Writing text in to a File
IN[3]
def main():
f = open('example1.txt', 'w')
f.write("Content to be entered into the file")
f.close()
f = open('example1.txt', 'r')
print(f.read())
if __name__ == "__main__" :
main()
OUT[3]
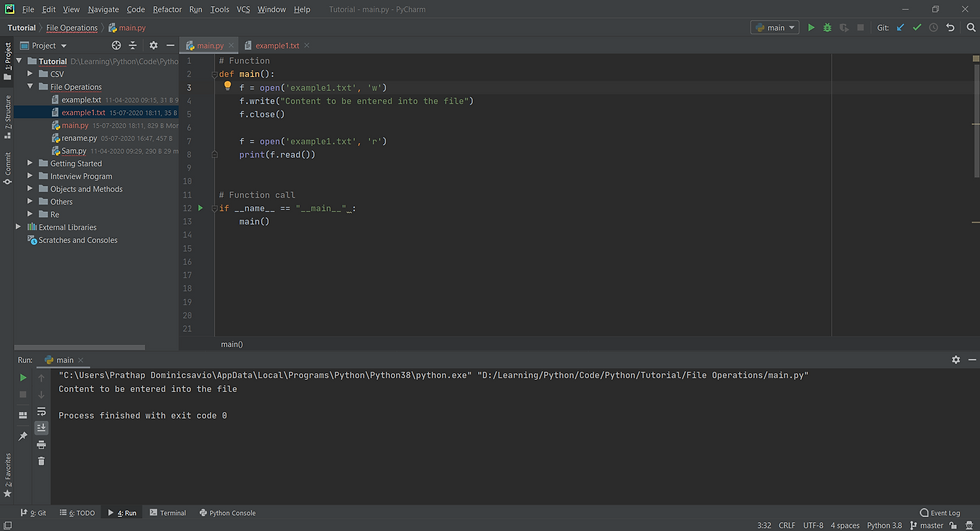
EXPLANATION
In above create section we would have created a txt file named example1, now let us write some data in it. To do that we have an another method named write(), write method takes a single argument and we have to pass our data as this argument.
Reading and Closing a File
IN[4]
def main():
f = open('example.txt', 'r')
print("Reading entire file\n", f.read())
print("\n Reading first 10 characters", f.read(10))
print("\n Reading a line", f.readline())
print("\n Reading multiple line", f.readlines())
f.close()
if __name__ == "__main__" :
main()
OUT[4]
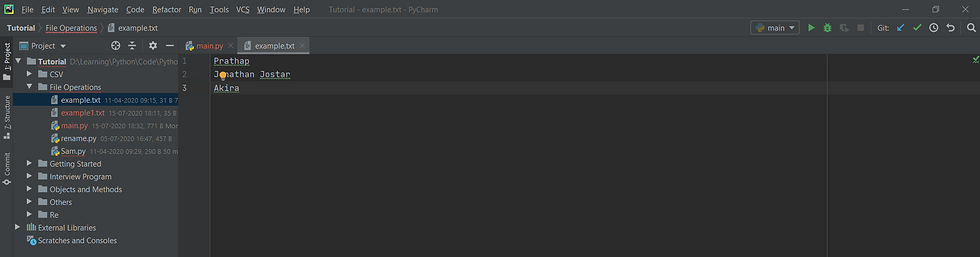
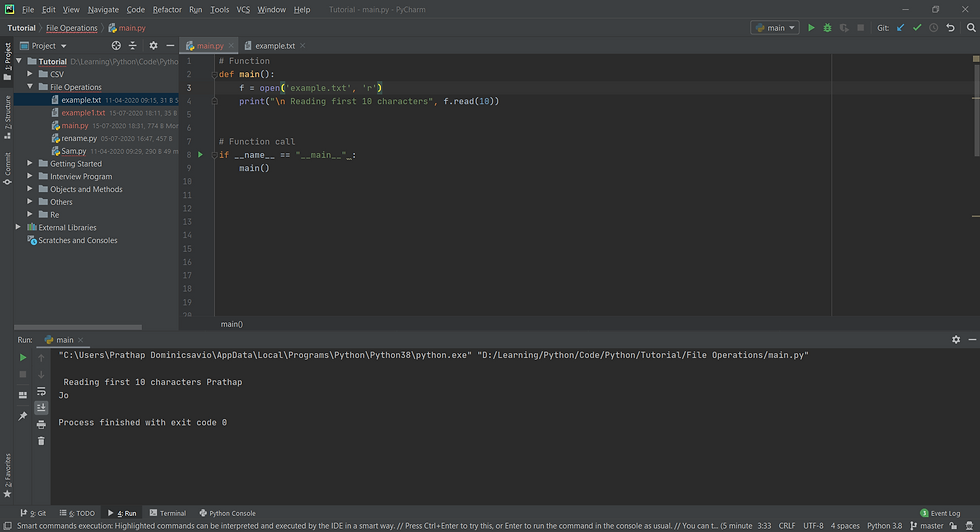
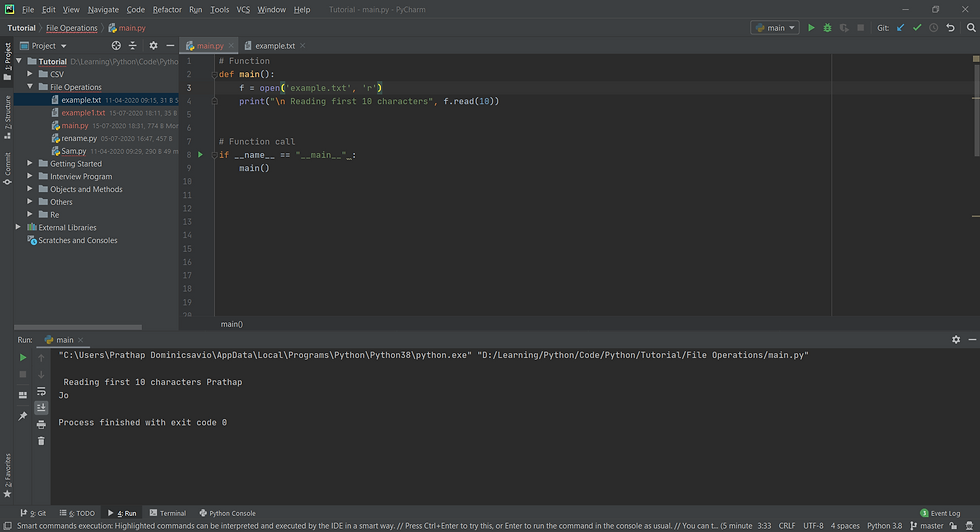
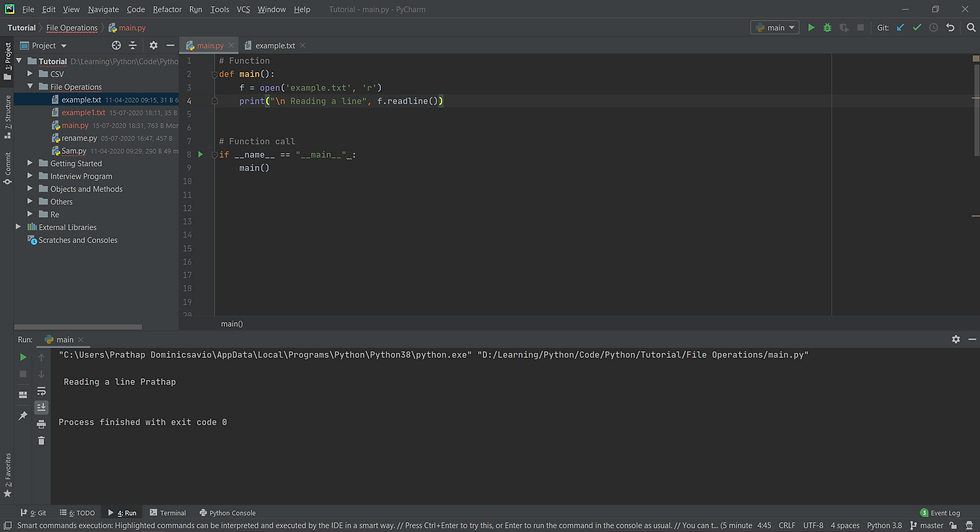
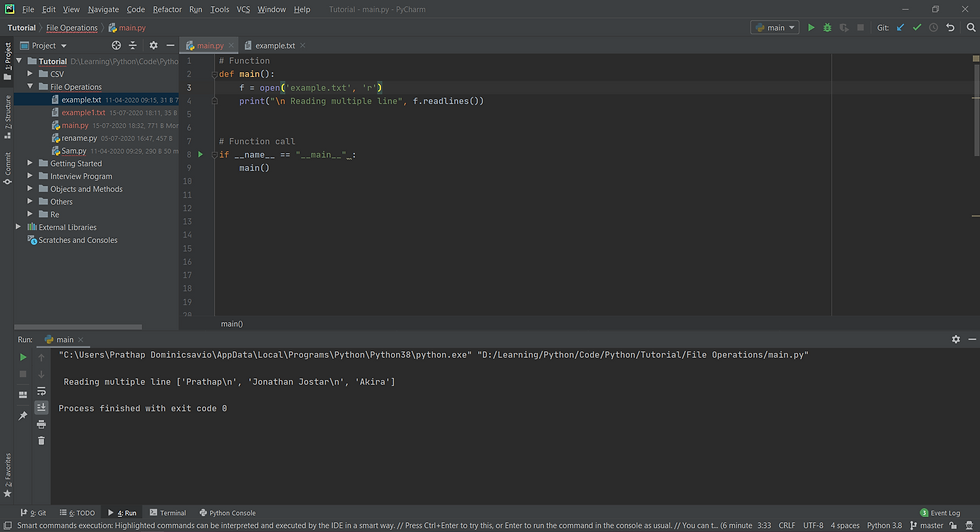
EXPLANATION
We use read() to read the entire file and by passing a argument(number) we can tell read method to read that number of character from a file. Similarly we pass readline to read first line and readlines to read multiple lines.
Deleting a File
IN[5]
import os
if os.path.exists("example1.txt"):
os.remove("example1.txt")
else:
print("The file does not exist")
OUT[5]
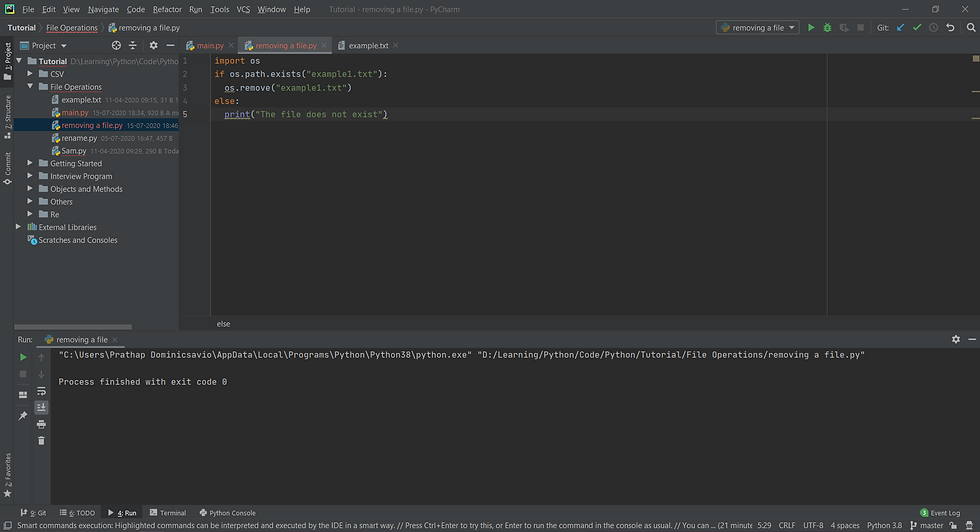
EXPLANATION
By using method from os package, os.path.exists("example1.txt") returns true if file is available and false is not and by using this conditional check we remove the file using remove() method if it exist.