In this post we will learn variables in Python, on any program we have to provide input or data so that our program compiles and provide output. This is where the variables comes to play, which holds our input for processing. Lets get started,
Variables are reserved memory location to store value, these variables support different data types from Numbers, List, Tuple, Strings to Dictionary, etc. Variables can be declared by any name or even alphabets.
Declaring Variable
As mentioned above to declare a variable just give name or alphabet with equal then value i.e like,
IN[1]
N = 10
Car = "Audi"
print("The memory address of N is", hex(id(N)))
print("The memory address of Car is", hex(id(Car)))
QUT[1]
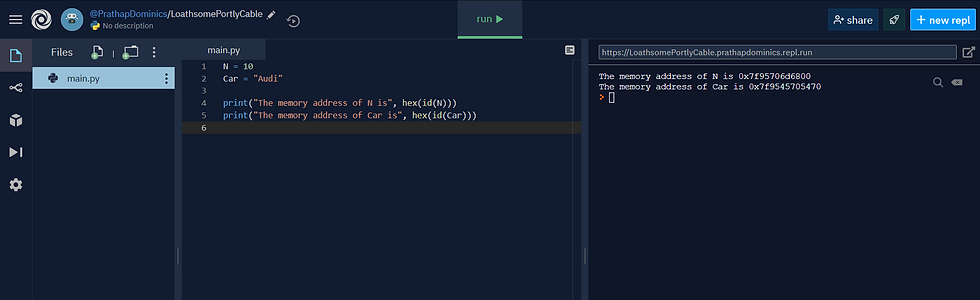
In the above program we can see there are two variables named N and Car which hold values 10 and "Audi". We can see their memory address by using the method id() and converting it to hex(), finally printing it using print() method.
Local and Global Variable
A variable can hold more than one value if it has been declared as a two separate variable type like Global or Local. Look at the below code,
IN[2]
f = 101
def vari():
f = "This is local variable"
print(f)
print(f)
vari()
OUT[2]
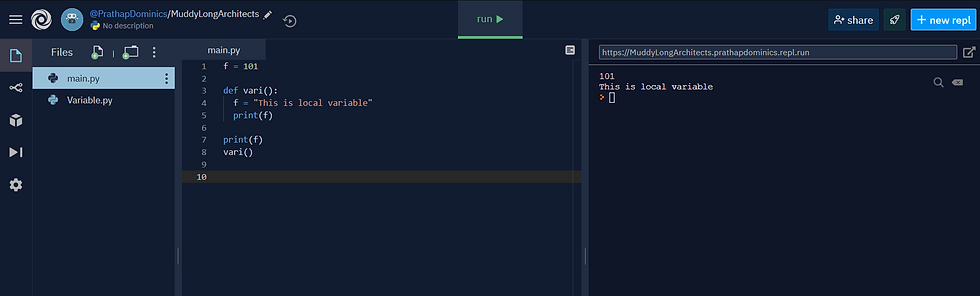
In above code f is declared and value 101 is stored which is declared globally, so whenever we print that variable f is will out the value 101 but the same variable f is re-declared again inside a function vari() as "This is local variable" this will only be available when the function vari() as this is local variable which is alive or accessible only inside that function.