In this post we will what are Booleans and how they are used across the python. Lets get started,
Booleans are one of the Datatypes in python which are used for conditional evaluation. They can hold only two values i.e, either True or False. Below we will learn how to use them.
IN[1]
print(5 == 5)
OUT[1]
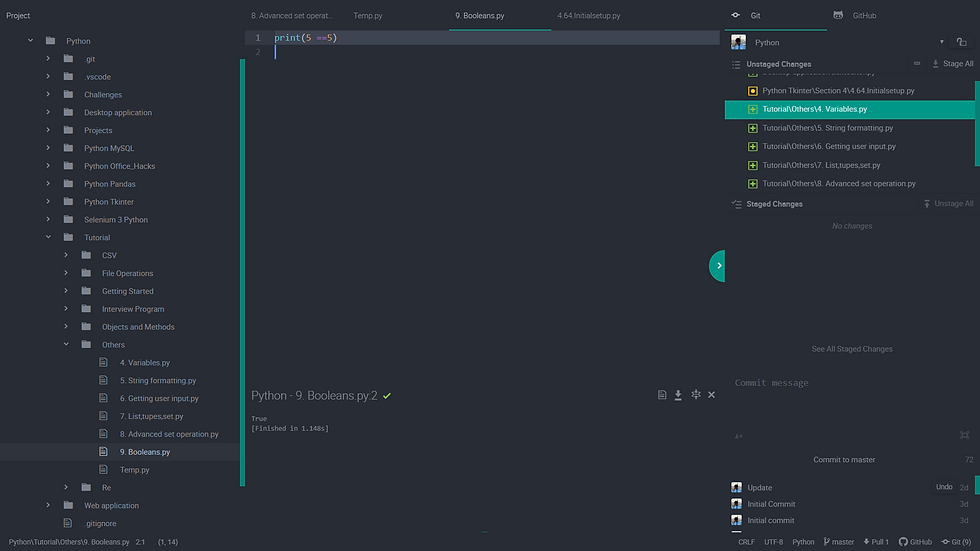
From above we can see that inside a print function we have an conditional statement or an expression i.e, 5 == 5. Since the expression 5 == 5 is actually correct and true. When we compile it, it will return True. Note single = is used to assign value and == is used to compare values.
IN[2]
a = (5 != 5)
print("The expression of 5 != 5 is : ",a)
a = (5 > 5)
print("The expression of 5 > 5 is : ",a)
a = ( 5 < 5)
print("The expression of 5 < 5 is : ",a)
a = (5 >= 5)
print("The expression of 5 >= 5 is : ",a)
a = ( 5 <= 5)
print("The expression of 5 <= 5 is : ",a)
OUT[2]
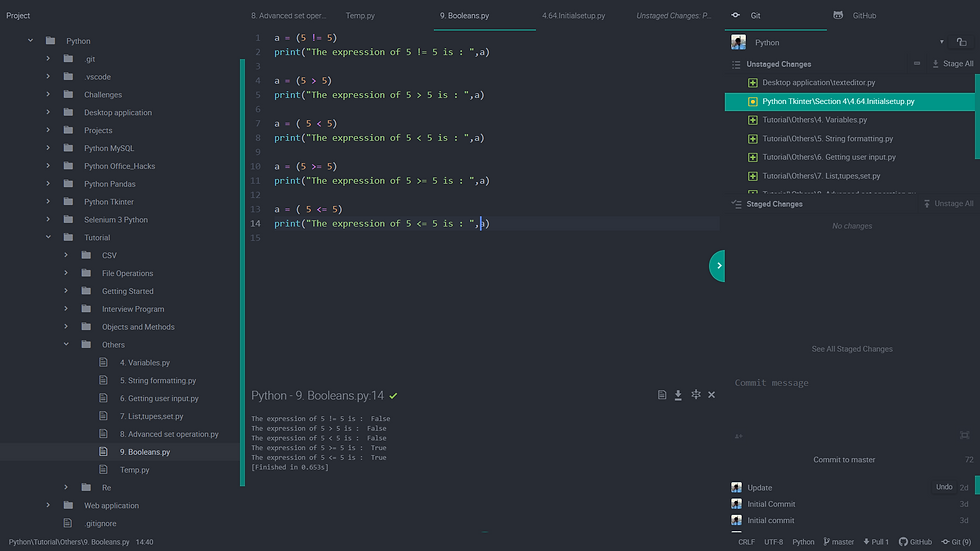
Similarly we can also assign this expression to a condition and then display it using print function.
IN[3]
friends = ['Rolf', 'Bob']
abroad = ['Rolf', 'Bob']
print(friends == abroad)
OUT[3]
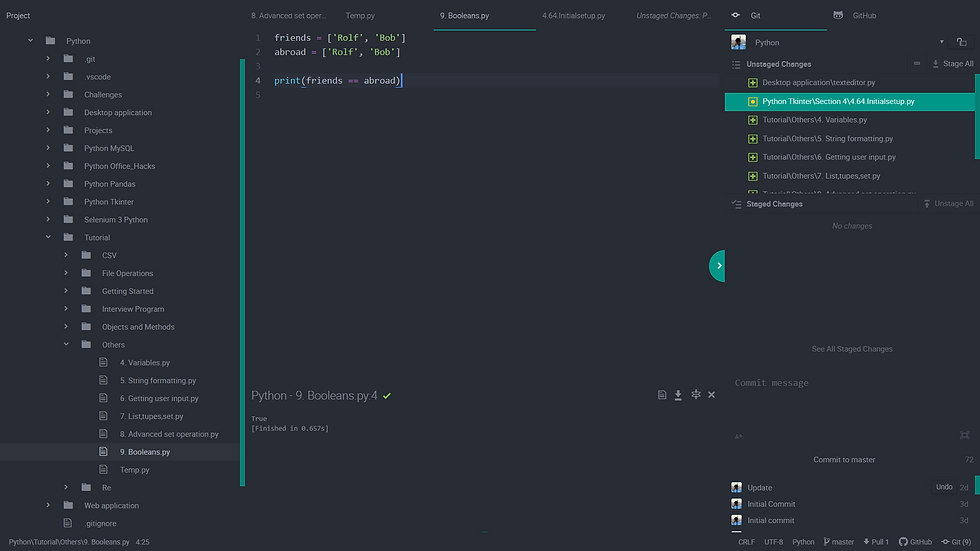
This way we can also evaluate and compare elements on the list, and return it to true if there are equal and false if not.
IN[4]
friends = ['Rolf', 'Bob']
abroad = ['Rolf', 'Bob']
print(friends is abroad)
OUT[4]
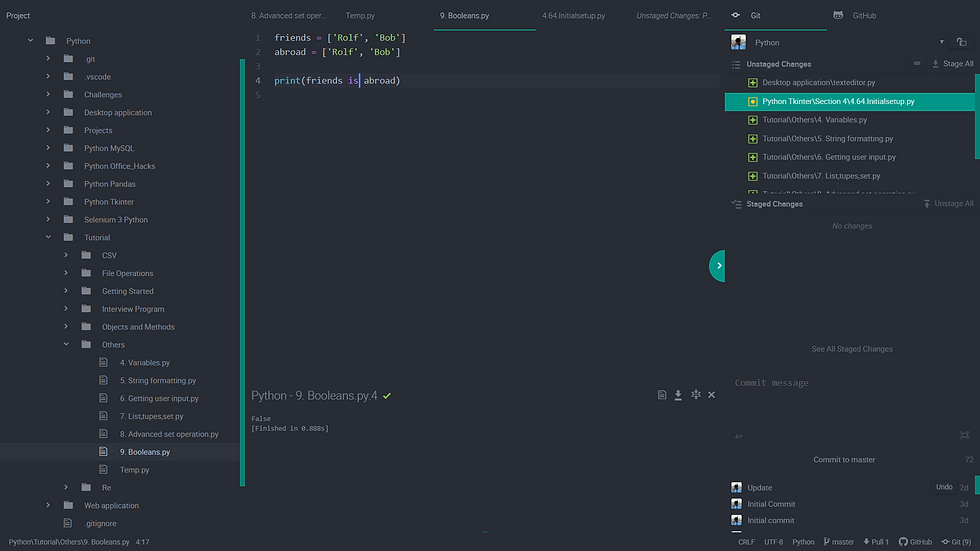
When comparing two list using keyword is, instead of comparing elements in the list it will compare two list. Although both the list has same element they are different(i.e, with difference variable, memory location and so on). So when we compare our two list it will return False.