In this post we will see Advanced Set Operations. Lets get started,
IN[1]
friends = {'Bob', 'Rolf', 'Anne'}
abroad = {"Bob", "Anne"}
local_friend = friends .difference(abroad )
print(local_friend)
OUT[1]
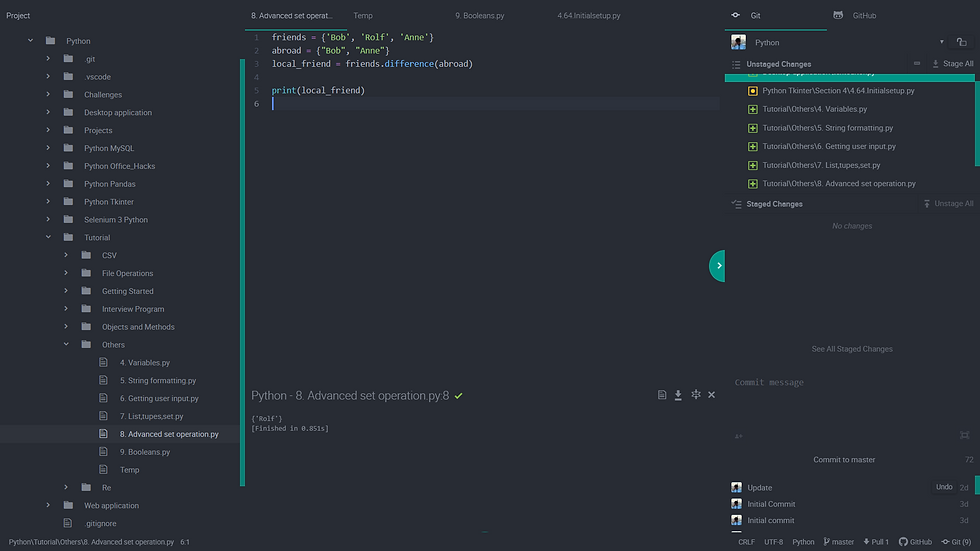
Set Difference, we can use difference() function to find out the odd element between the Set. To do that declare or define in the following format,
Set_1.difference(Set_2)
Set_1 has to be the set for which the difference if evaluated with the help of Set_2. From our above example we will get 'Rolf' since Set_2 has all the elements of Set_1 except 'Rolf'.
IN[2]
friends = {'Bob', 'Rolf', 'Anne'}
abroad = {"Bob", "Anne"}
local_friend = abroad.difference(friends)
print(local_friend)
OUT[2]
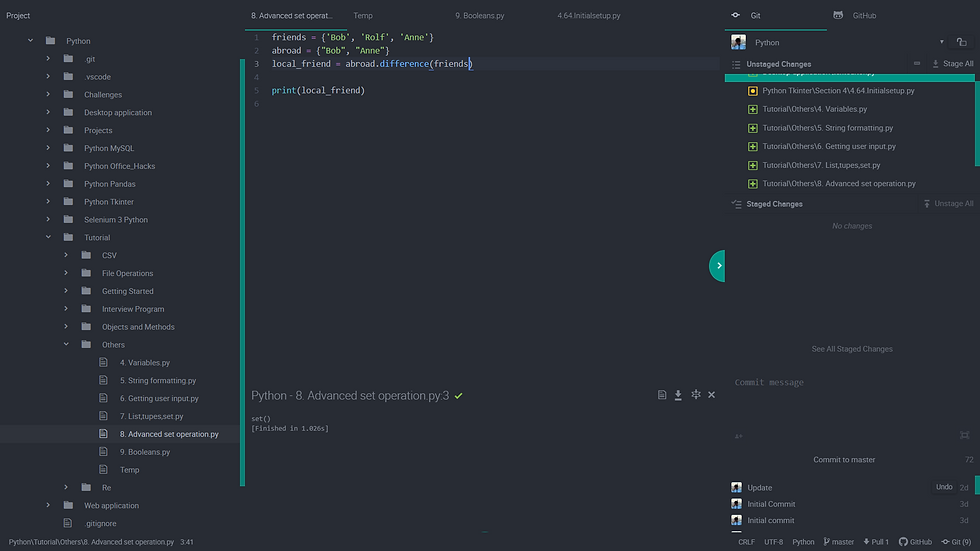
From our above example we can see that the set abroad has all the elements of friends and return a empty set. In python set() notation is returned when denoting a empty set.
IN[3]
local = {"Rolf"}
abroad = {"Bob", "Anne"}
friends = local.union(abroad)
print(friends)
OUT[3]
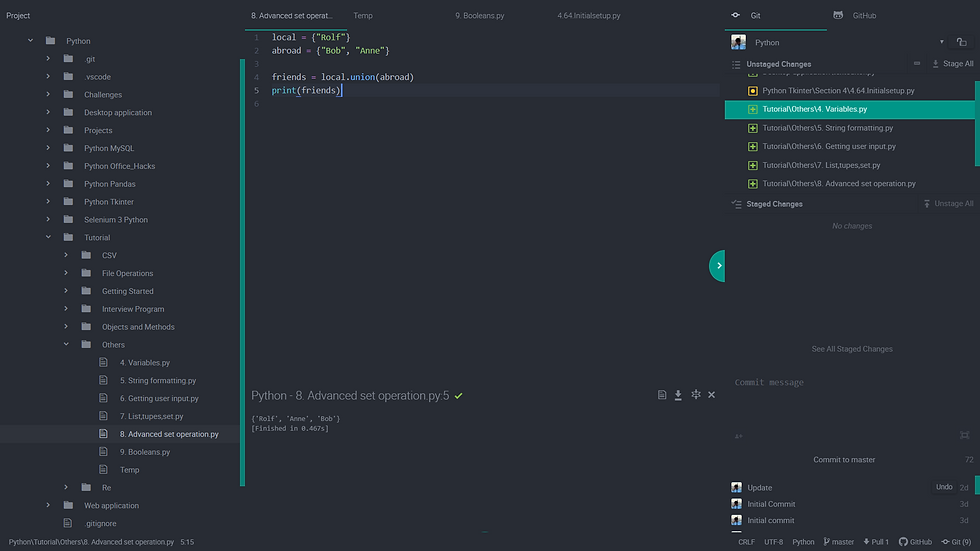
We can add an element into a set using Union() method. To do that follow the below syntax,
Set_1.union(Set_2)
This will combine Set_1 with the Set_2 and since duplicated are not allowed in Sets only one element will be present after the union of these sets if sets were have common element.
IN[4]
art = {"Bob", 'Jen', 'Rolf', 'Charlie'}
science = {'Bob', 'Jen', 'Adam', 'Anne'}
both = art.intersection(science)
print(both)
OUT[4]
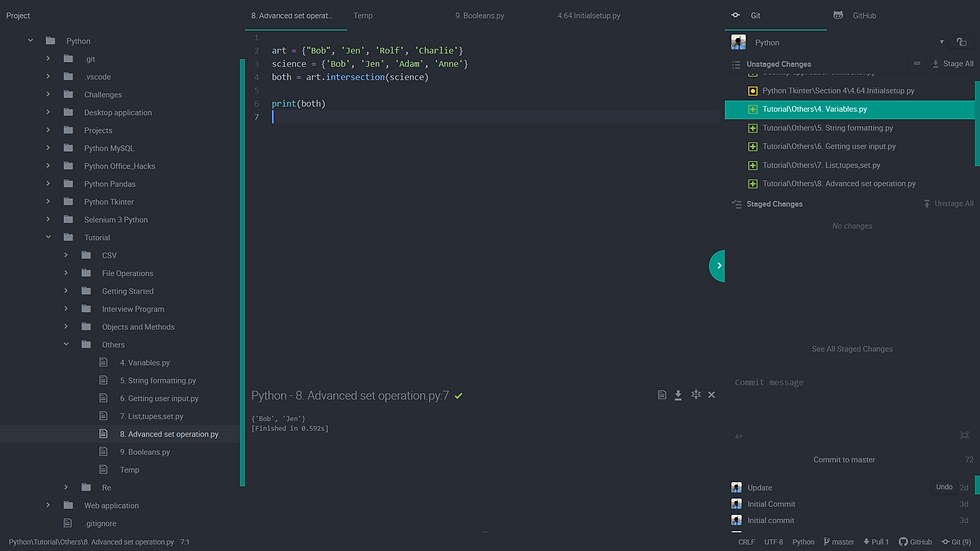
The set interaction function will only display elements which are common on the both the sets. We can store it in a variable to use them or you can directly pass it to print function to display it.