In this post we will write our first Tkinter program, we will be building a simple window with single label printing our name in it. From this and coming post we will first see code, code output then its explanation. Lets get started,
IN[1]
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
ttk.Label(root, text = "Prathap Dominicsavio", padding = (50, 30)).pack()
tk.mainloop()
OUT[1]
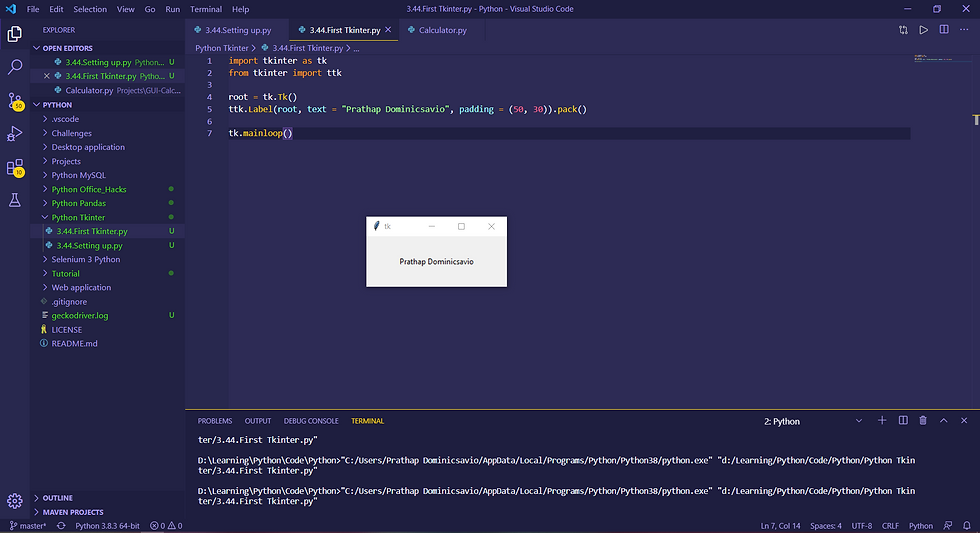
EXPLANATION
Firstly, import tkinter as tk Since we use tkinter module we import them into our program and provide it with an alias tk. As mentioned earlier we use Label, Labels comes under the category of widgets in ttk module. In order to import then into our program we write from tkinter import ttk.
Now to create a window, since we are going to create a single master window we declare a variable named root and call a method named Tk() as this root = tk.Tk(). Simply creating a root window will not display a window when program is ran, in order for window to run we must call mainloop method like tk.mainloop(). If program is ran now the python interpreter will stop at the mainloop until the program is closed i.e, the program window can still be closed as window has native window operations as shown below.
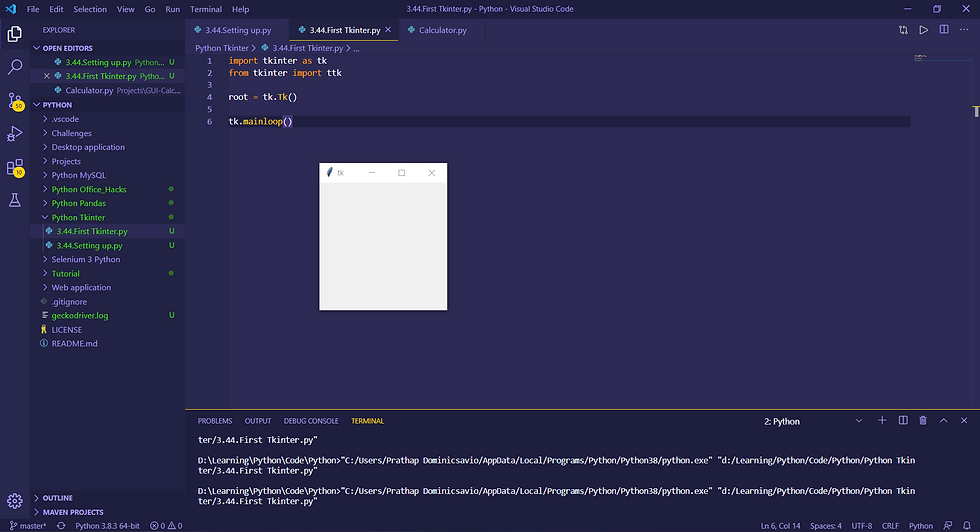
Next comes the label part, we use Label method of ttk on which we will be passing the following attributes i.e,
ttk.Label(root, text = "Prathap Dominicsavio", padding = (50, 30)).pack()
Mentioning on which window should this label on, in our program it is root.
Display text, in our program it is our names.
Height and Width of the window, in our program it is 50px and 30px.
Then the Pack method which we will be discussing in detail on the upcoming posts.