On our earlier articles we might have learnt how to download and setup the environment for practicing selenium. In this one we will teach you how to run your first selenium code.
Downloading selenium and its jar
You need certain executable and Jar file for compiling and running selenium code. Follow the below steps to download them,
1. Download selenium webdriver for our google chrome from this link https://chromedriver.storage.googleapis.com/index.html?path=77.0.3865.40/
2. Download selenium jar file from the following link https://bit.ly/2zm3ZzF
Importing the selenium into our project
1. Open your Intellij and open a new Java Project. Enter your project name and leave everything else to default settings.
2. Once you have opened you project click File ---> Project Structure ---> Add button
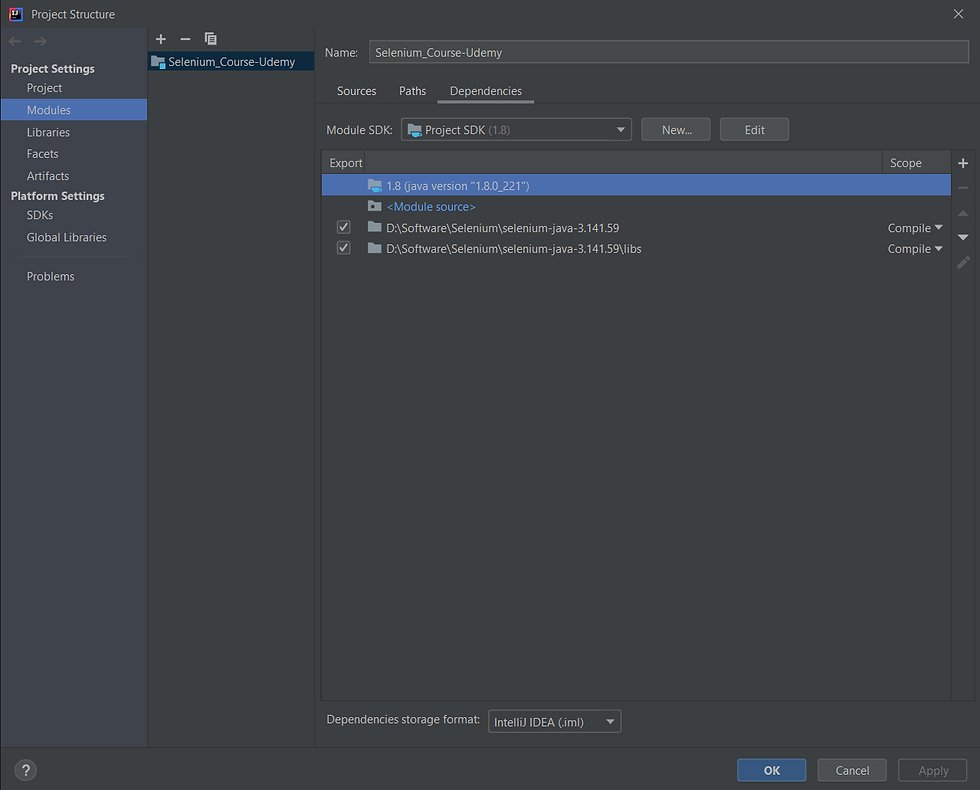
3. The select and redirect your path to the download jar file and click apply.

4. And click apply to add them to you project. Now create a new class file by clicking the Project directory ---> New ---> class and enter the class name.
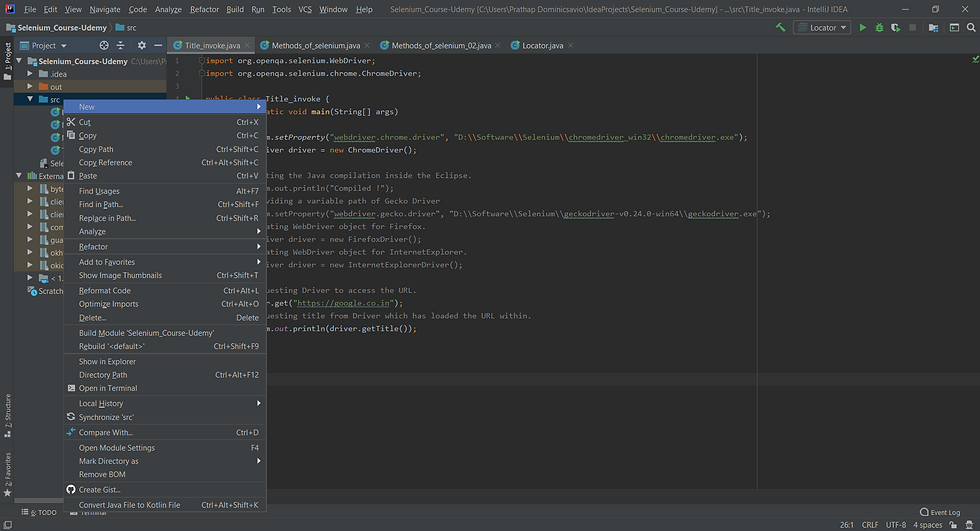
5. Now enter the below attached code, and press the shortcut keys Ctrl+Shift+F10 to run the code. this below snippet will open and gets the google webpage's tile and print it in the output tab.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class Title_invoke {
public static void main(String[] args)
{
System.setProperty("webdriver.chrome.driver", "D:\\Software\\Selenium\\chromedriver_win32\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("https://google.co.in");
System.out.println(driver.getTitle());
}
}
Code Explanation :
import org.openqa.selenium.WebDriver
This is for importing selenium classes and methods into our code.
import org.openqa.selenium.chrome.ChromeDriver
This for invoking the selenium jar which we have downloaded earlier.
System.setProperty("webdriver.chrome.driver", "D:\\Software\\Selenium\\chromedriver_win32\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
This will allow to us to call forth the drivers for opening the Google chrome.
driver.get("https://google.co.in");
This will tell the drivers to open the google on google chrome.
System.out.println(driver.getTitle());
This will print the title name of the google in the Intellij output tab.
I hope this above code could have given you a slight hint on how to run a selenium code to get and test information from web application.