An effective practice of writing program is to make few changes for every data from users, if we hardcode the input then we have to make change in program every time there is a change in input. To avoid this we have to get input from the user dynamically.
In Python getting input and printing them out is relatively easy with input and print functions. Note that for python version 2x we have to use raw_input function. Lets get started,
IN[1]
input()
Input function in Python 3
'Input function in Python 3'
OUT[1]
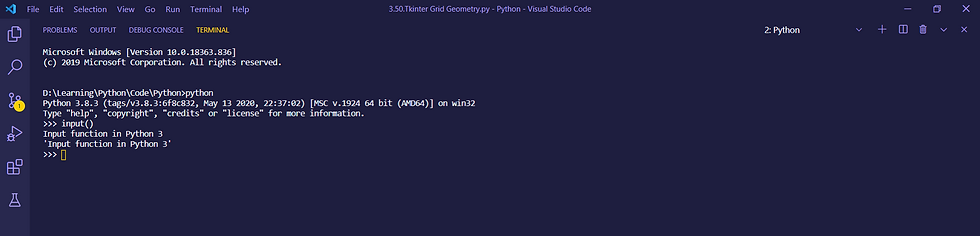
The interpreter will stop at the input function to get the input from the users and will only resumes after hitting enter key. Input function will take value and it does not have timeout nor character limit.
IN[2]
v = input()
Hell
b = input()
22
print(type(v))
print(type(b))
OUT[2]

The default datatype of the value stored from input function is always string, despite passing number or string into datatype. From above snap we could see variable v has "Hell" stored which is string and variable b has "22" is number but when you use type method to see its datatype you will see it has been stored as an string.
IN[3]
v = int(input())
22
print(type(v))
OUT[3]

But the above mentioned problem can be overcome from using type casting.