In this post we will learn how to run external command using Subprocess module in python. This Subprocess module is extremely useful when we want run external command and capture those output. This is a little complicated topic so we will try to keep simple as much as possible. Lets get started,
To run a command, first we need to import Subprocess module and then use the Subprocess.run method. Lets now try to get the list of files in the directory. To do that,
IN[1]
import subprocess
subprocess.run('dir')
OUT[]
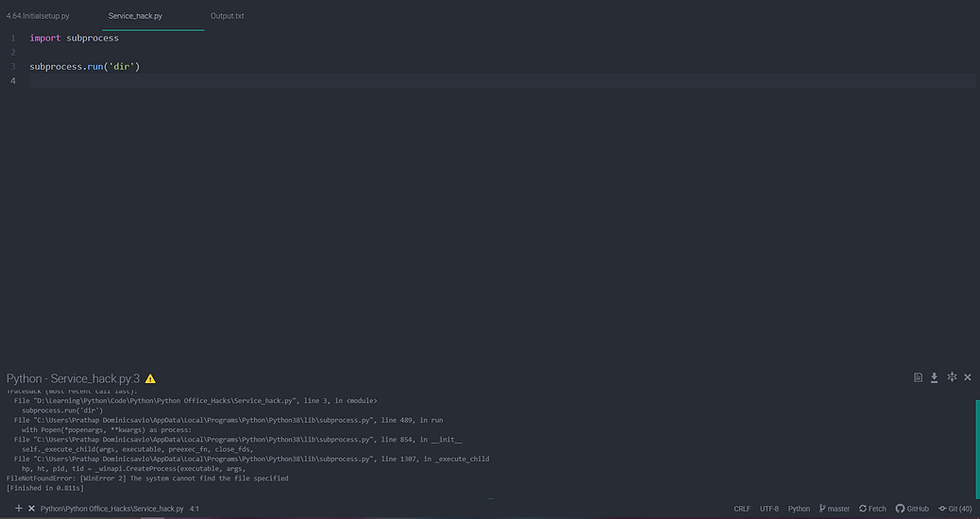
At this point we would have gotten a "File not Found Error" this is because "dir" command is build within shell. To avoid this problem we have to insert an another argument named shell and set it to True.
IN[2]
import subprocess
subprocess.run('dir', shell = True)
OUT[2]
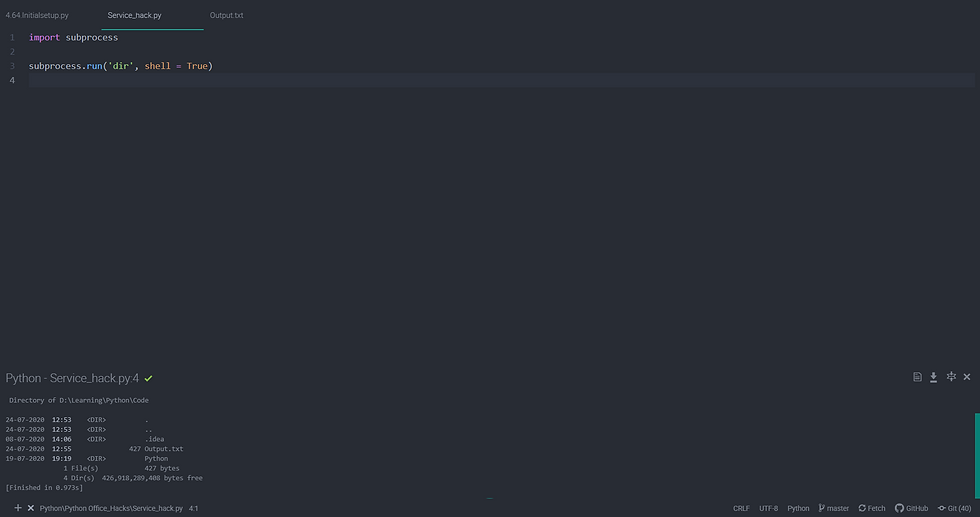
When shell = True, we can run the entire command including the argument as an string. This might come as an security hazard so please avoid untrusted arguments. You might have noticed that the subprocess is printing everything as a standard print statement in python and this because standard output is not captured and the output goes to the shell.
To avoid this and capture it to a variable we can pass in on a capture argument inside run method and save it in a variable.
IN[3]
import subprocess
p1 = subprocess.run('dir', capture_output = True, shell = True)
print(p1)
OUT[3]

Now you can see that the variable named p1 has the output of the shell command stored but problem is that the value stored in the variable are in bytes so we might have to decode them. There is also an another in which we can pass in an argument on the run method text to store the value as string directly to the variable it is assigned. Both methods has been shown below.
IN[4]
import subprocess
p1 = subprocess.run('dir', capture_output = True, shell = True)
print(p1.stdout.decode())
p2 = subprocess.run('dir', capture_output = True, shell = True, text = True)
print(p2.stdout)
OUT[4]
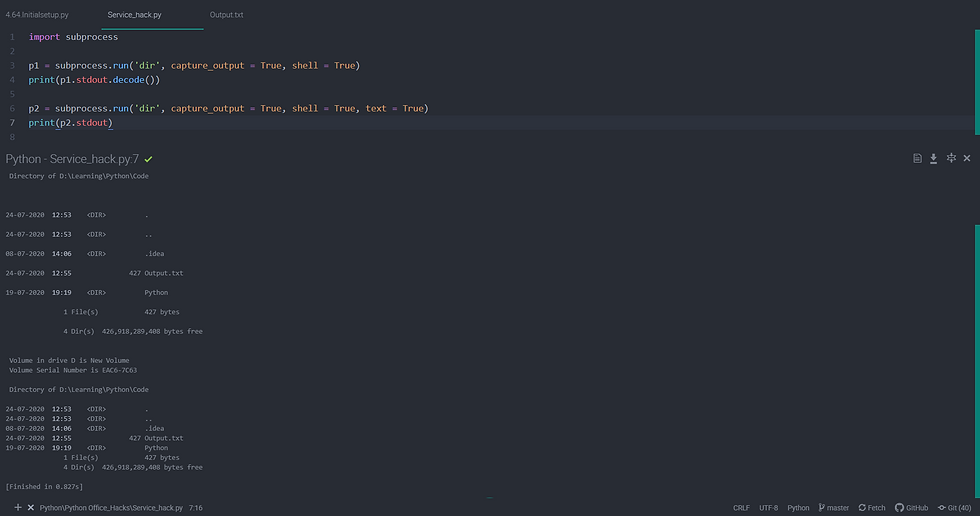
P