In this post we will write our first project, an Greeting Application which will get our name from windows and print it in the console. From this project we will also learn about the widgets named Entries. Lets get started,
IN[1]
import tkinter as tk
from tkinter import ttk
def greet():
print(f"Welcome, {name_entry.get() or 'World'}")
root = tk.Tk()
user_name = tk.StringVar()
name_label = ttk.Label(root, text = "Name : ")
name_label.pack(side = "left", padx = (0, 10))
name_entry = ttk.Entry(root, width= 30, textvariable = user_name)
name_entry.pack(side = "left", expand = True)
name_entry.focus()
greet_button = ttk.Button(root, text = "Greet", command = greet)
greet_button.pack(side = "left", fill = "x", expand = False)
quit_button = ttk.Button(root, text = "Quit", command = root.destroy)
quit_button.pack(side = "left", fill = "x", expand = False)
tk.mainloop()
OUT[1]
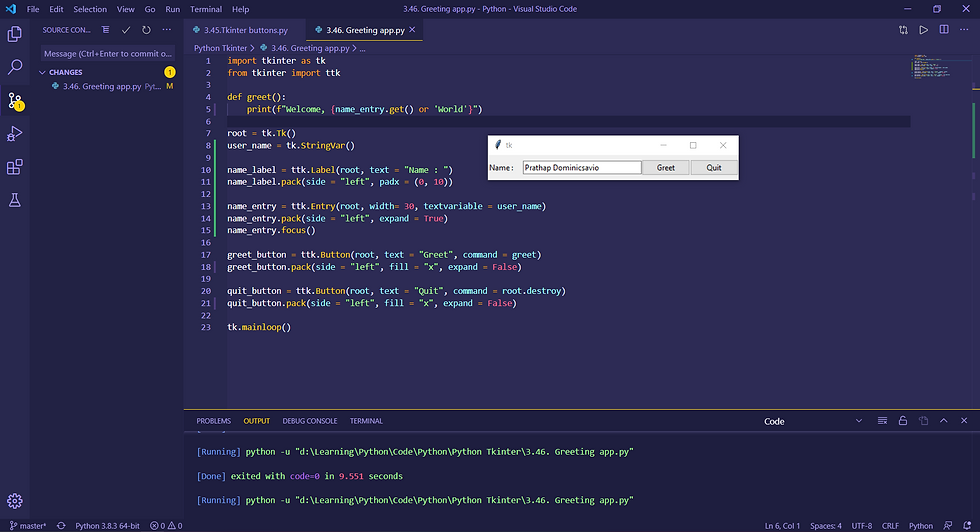
EXPLANATION
First we will create a label for holding the string named Name.
name_entry = ttk.Entry(root, width= 30, textvariable = user_name)
name_entry.pack(side = "left", expand = True)
name_entry.focus()
Then we will create entry which will provide a text box for getting the input from the users. It has following parameters i.e,
width, to provide the text field of desired width.
textvariable, to store the value entered in the text box.
Finally we use the focus() method to move the cursor directly to the text box when the window is opened. If you notice parameter in the entry textvariable has a variable name passed, so don't forget to create a variable as Stringvar().
f"Welcome, {name_entry.get() or 'World'}"
In the above program we have used a F string inside a greet function, this will print the entered named and if no string is entered then raw string 'World' will be printed.
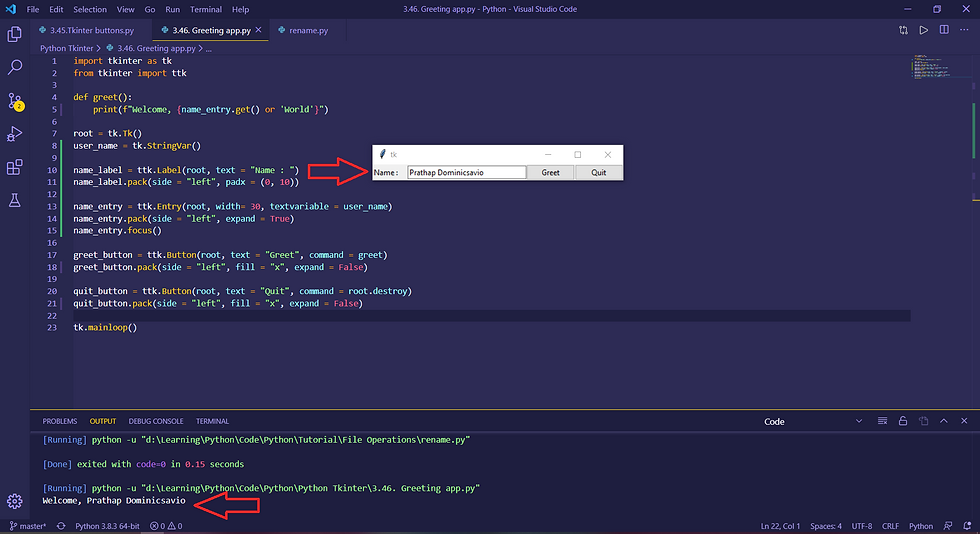