In this post we will learn about Tkinter buttons on how to use them and tweak them based on our requirements. We will also learn about positioning of theirbuttons so that we can understand how buttons alignment are treated by Tkinter. Lets get started,
IN[1]
import tkinter as tk
from tkinter import ttk
def greet():
print("Welcome, Prathap Dominicsavio")
root = tk.Tk()
greet_button = ttk.Button(root, text = "Greet", command = greet)
greet_button.pack(side = "left", fill = "x", expand = True)
quit_button = ttk.Button(root, text = "Quit", command = root.destroy)
quit_button.pack(side = "left")
tk.mainloop()
OUT[1]
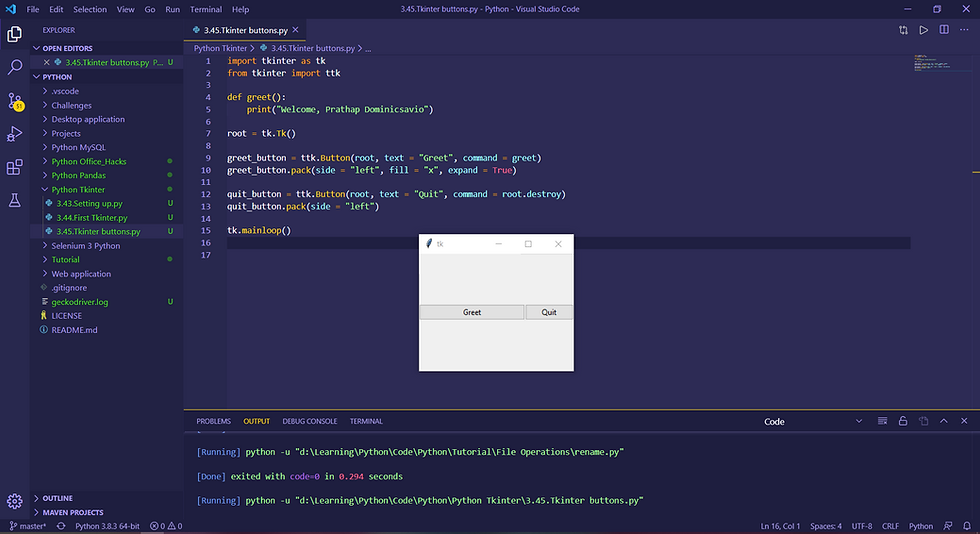
EXPLANATION
Firstly, we want to perform certain action when a button is pressed similarly when we press our button it will print welcome along with our name. It has been defined inside a function greet() which will be invoked when our button is pressed.
we can create our button using the function Button(), it has following parameters i.e,
greet_button = ttk.Button(root, text = "Greet", command = greet)
greet_button.pack(side = "left", fill = "x", expand = True)
Mentioning on which window should this button on, in our program it is root.
Display text, in our program it is our button name.
Command, the function name has to be passed (without parenthesis) so that when button is pressed this function will be called.
Then the Pack method which we will be discussing in detail on the upcoming posts, but now we will see the use of parameters used in our current program.
Side, at which position the button should be placed
Fill, what positioning does the button has to be filled like vertical or horizontal.
Expand, whether the button has to be expanded when the window is expanded.
quit_button = ttk.Button(root, text = "Quit", command = root.destroy)
quit_button.pack(side = "left")
We will create an another button which will close the window when the button is pressed.
To do that we will use destroy() method along the the window object root so that it will destroy root window.
Now well will perform little experiments to understand all the function of the button mentioned above,
I. Now Side is assigned as Right, first Greet button will be at right since it is declared and assigned first then the Quit button will be at right to the Greet button. In Tkinter when widgets are declared a dedicated vertical or horizontal space will be allotted. By default Side is Top.
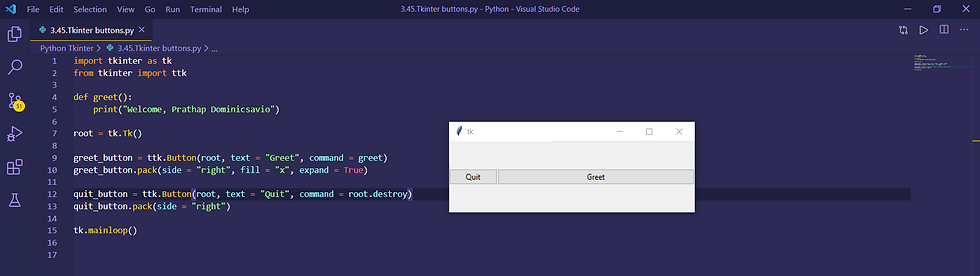
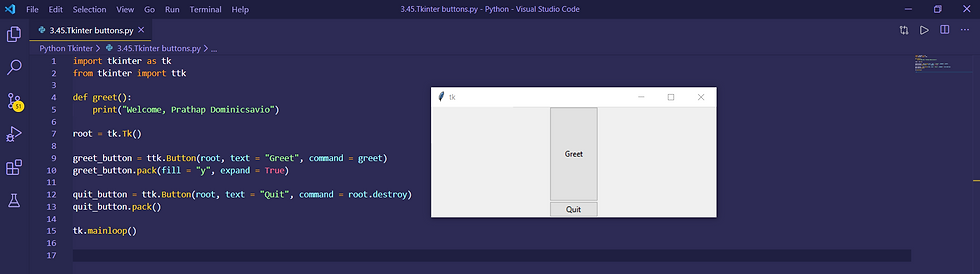
II. Expand is False, now even when the window is expanded the button will stay at the default width and wont extend along with the window.
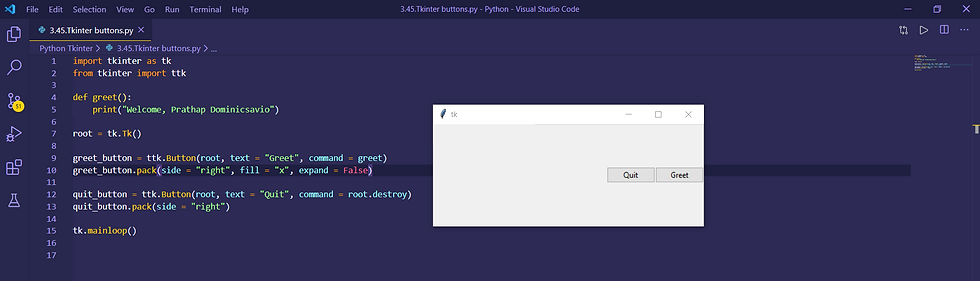
III. Fill is y and Expand is True. If you expand the window the button will fill in the vertically as the fill is y.
