In Tkinter, every widgets has its own Style Class associated with it. Thus providing the flexibility of changing the themes of individual widgets in the tkinter application. Let us first see how to find those classes.
IN[1]
import tkinter as tk
from tkinter import ttk
try :
from ctypes import windll
windll.shcore.SetProcessDpiAwareness(1)
except:
pass
root = tk.Tk()
root.geometry("300x50")
style = ttk.Style(root)
label = ttk.Label(text = "Hello")
label.pack()
print(label["style"])
root.mainloop()
OUT[1]
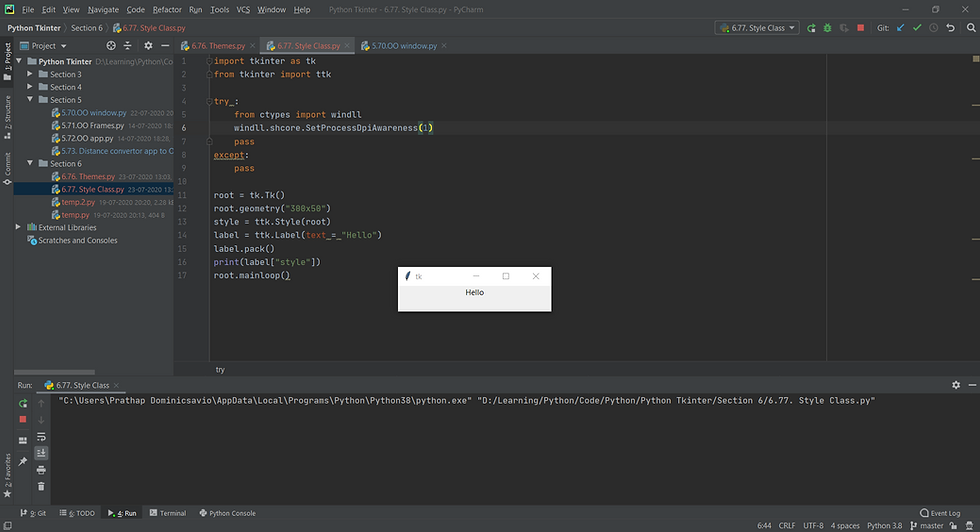
Well the print statement didn't print anything as it was using default style and this begs the question what is the default style to find out that using the winfo method as shown below.
IN[2]
import tkinter as tk
from tkinter import ttk
try :
from ctypes import windll
windll.shcore.SetProcessDpiAwareness(1)
except:
pass
root = tk.Tk()
root.geometry("300x50")
style = ttk.Style(root)
label = ttk.Label(text = "Hello")
label.pack()
print(label.winfo_class())
root.mainloop()
OUT[2]
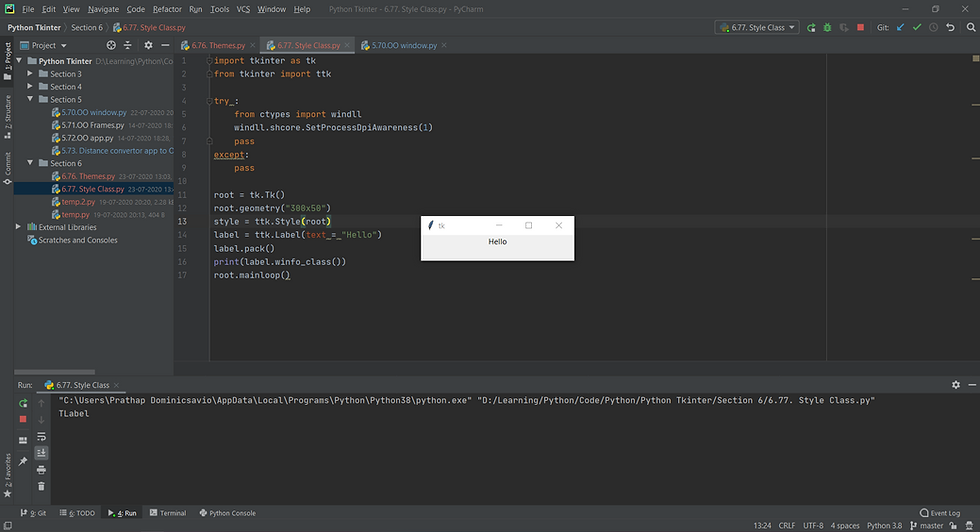
Styles are even applied on widgets which are not applied on the window or application. Below we have an code where the entry widgets don't have pack on them and so it won't be displaying its widgets in the window but by using winfo method we can get it current Style class.
IN[3]
import tkinter as tk
from tkinter import ttk
try :
from ctypes import windll
windll.shcore.SetProcessDpiAwareness(1)
except:
pass
root = tk.Tk()
root.geometry("300x50")
style = ttk.Style(root)
label = ttk.Label(text = "Hello")
entry = ttk.Entry(width = "10")
label.pack()
print(entry.winfo_class())
root.mainloop()
OUT[3]
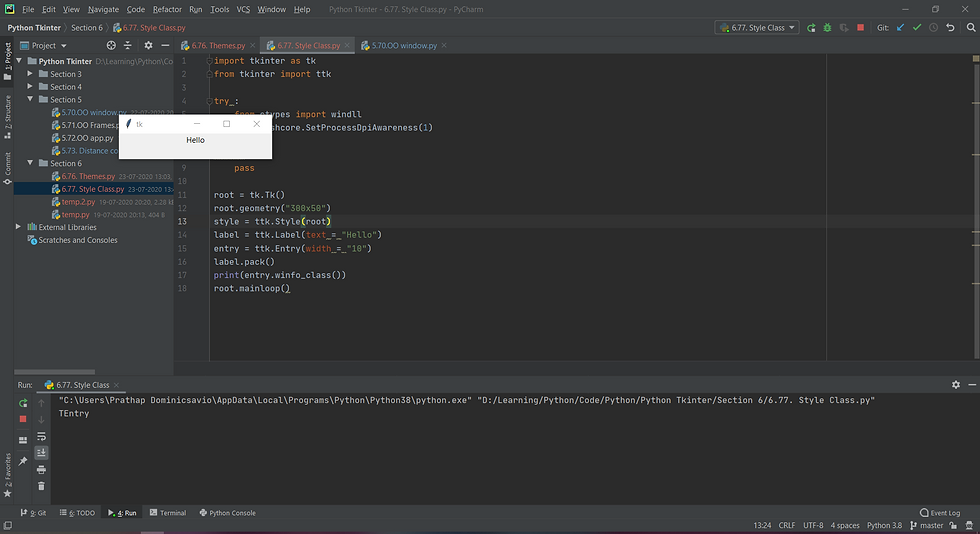
If you notice you will find all the Class names of Styles starts with 'T' like Tlabel, Tentry ..etc. Well most of the Tkinter Style classes start with unless there are T themselves.