In this post we will see how to introduce scales onto our Tkinter application. Tkinter allows us to place both vertical and horizontal scales. Like Dropbox and checklist, scales provide input when slider is moved. Each position hold a numeric value with which we can evaluate conditions if required. Lets get started,
IN[1]
import tkinter as tk
from tkinter import ttk
from PIL import Image, ImageTk
from ttkthemes import ThemedStyle
try :
from ctypes import windll
windll.shcore.SetProcessDpiAwareness(1)
except :
pass
root = tk.Tk()
root.title("Scales")
root.geometry("600x400")
root.resizable(False, False)
root.wm_iconbitmap('D:\Learning\Python\Code\Python\Desktop application\Bikini.ico')
style = ThemedStyle(root)
style.set_theme('scidgrey')
def handle_scale_change():
print(scale.get())
current_value = tk.IntVar()
scale = tk.Scale(root, orient = "horizontal", from_ = 0, to = 10, command = handle_scale_change, variable = current_value).pack(fill = "x")
root.mainloop()
OUT[1]
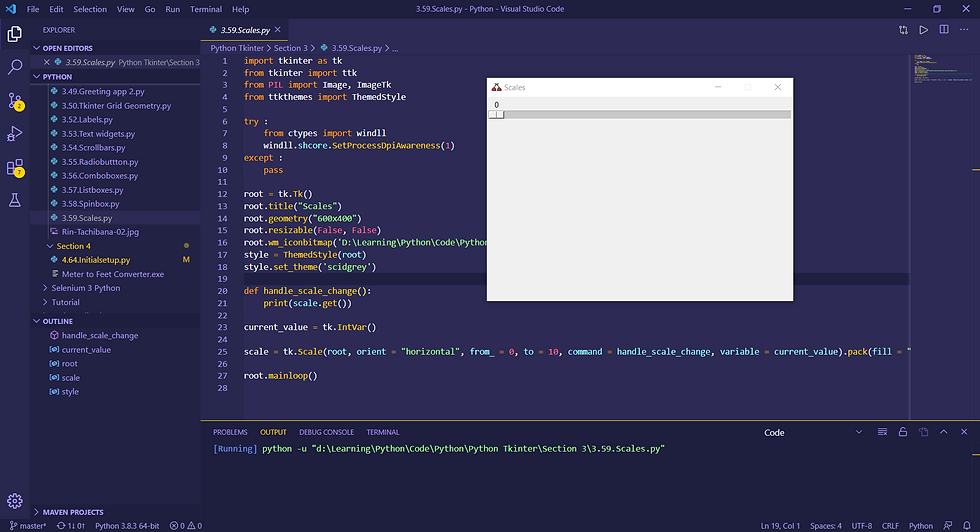
EXPLANATION
To define a scale, use a Scale class and passing the following arguments for the scale properties,
orient : Decides whether the widgets stays horizontal or Vertical.
from_ : Start value of the Slider. Note that the argument variable name is from_ not from as from is a reserved keyword in Python.
to : End value of the Slider.
command : Used for calling the method when this widget is interacted.
variable : Used for getting a value and store it in a variable.
Finally we pack us scale using Pack class. Whenever the slider in the scale is moved the function handle_scale_change is called which will print current value of slider.