In this section we will learn about spinboxes in Tkinter application. We will see how to use them and play with the other spinbox proprieties. Lets get started,
IN[1]
import tkinter as tk
from tkinter import ttk
from PIL import Image, ImageTk
from ttkthemes import ThemedStyle
try :
from ctypes import windll
windll.shcore.SetProcessDpiAwareness(1)
except :
pass
root = tk.Tk()
root.geometry("600x400")
root.resizable(False, False)
root.title("Spinbox")
root.wm_iconbitmap('D:\Learning\Python\Code\Python\Desktop application\Bikini.ico')
style = ThemedStyle(root)
style.set_theme('scidgrey')
initial_value = tk.IntVar(value = 20)
#spin_box = ttk.Spinbox(root, from_ = 0, to = 30, textvariable =initial_value, wrap=True).pack()
spin_box = ttk.Spinbox(root, values = (10, 20, 30, 40, 50), textvariable =initial_value, wrap=True).pack()
root.mainloop()
OUT[1]
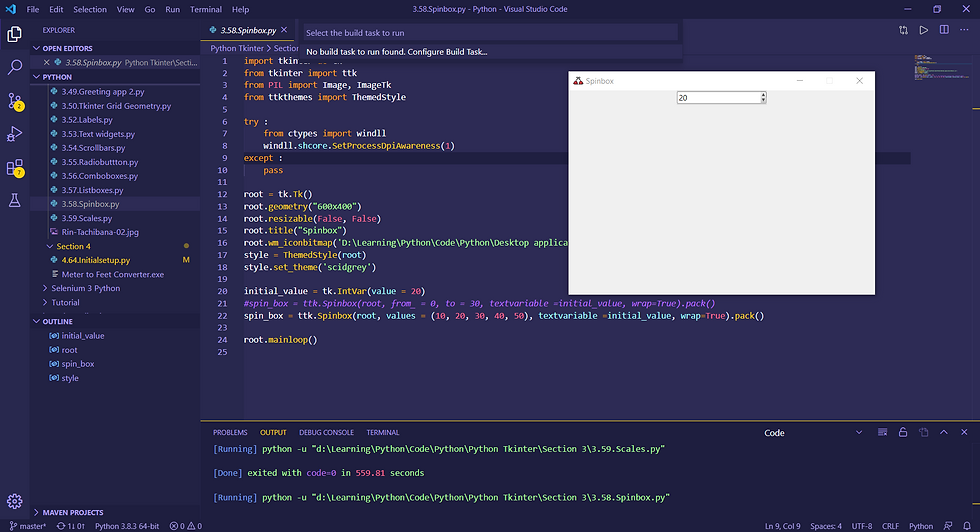
EXPLANATION
Unlike the Sliders, spinbox changes its value only when the up and down arrow button is pressed. If you look closely there are few thing to consider like start and end value along with the interval on which the value has to be increased or decreased.
To define spinbox in tkinter define a Spinbox class to a variable, following are its argument and properties.
from_ : Used to define START value of spinbox cycle.
to : Used to define END value of spinbox cycle.
values : If you wish to define a list of variable instead of from_ and to we use value argument.
textvariable : Usually used to define the start value and to store value as a variable.
When packed the value of the spinbox changes for every spin based on the interval defined, in our case 10 from 10 to 50 for every click.