In this post we will learn about Listboxes in Tkinter. We will learn how to use them as well as how to retrieve the selected value when a list option is selected.
IN[1]
import tkinter as tk
from tkinter import ttk
try :
from ctypes import windll
windll.shcore.SetProcessDpiAwareness(1)
except :
pass
root = tk.Tk()
root.title("Listboxes")
root.geometry("600x400")
root.resizable(False, False)
programming_languages = ("C", "Go", "Perl", "Javascript", "Python", "Rust")
langs = tk.StringVar(value = programming_languages)
langs_select = tk.Listbox(root, listvariable=langs, height=6)
langs_select["selectmode"] = "extended" #browse
langs_select.pack()
def handle_selection_change(event):
selected_indices = langs_select.curselection()
for i in selected_indices:
print(langs_select.get(i))
langs_select.bind("<<ListboxSelect>>", handle_selection_change)
root.mainloop()
OUT[1]
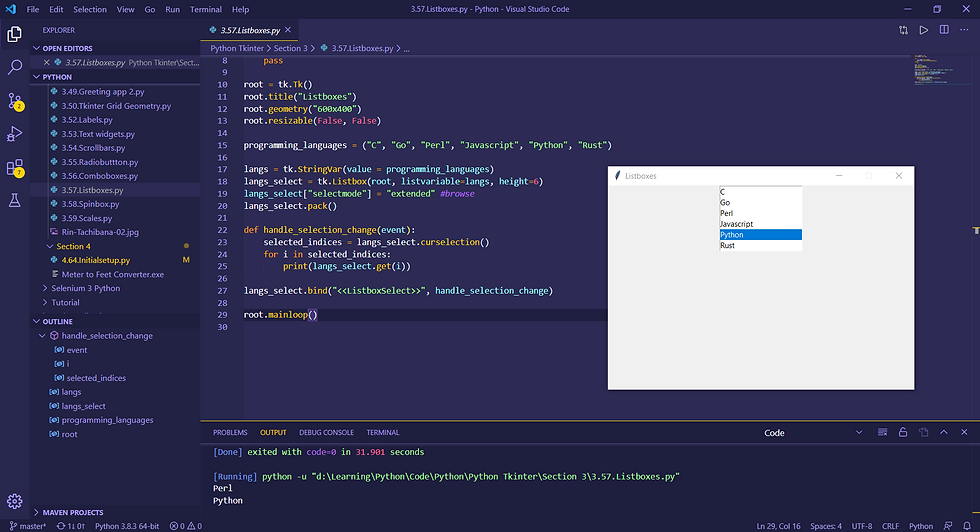
EXPLANATION
As we are working with listboxes which will be listing a list of option when clicked. We need to create a variable and pass in all the values as list. These will be over listbox options, next define a listbox using the class Listbox which has the below show attributes,
listvariable : Variable, used to store the value selected in the list.
height : Determine the standard display height of the list when expanded.
If your list is large and if you define a height much shorter than the list then the intended list will not be displayed fully. To avoid that and introduce the scroll inside the expanded list we have to set selectmode of the listbox variable to browse.
In order to get the value of the selected list then we have to bind the action of this selected event with the function. To do that we have to follow the below syntax,
listbox_variable.bind("<<ListboxSelect>>", function_name)
There is a catch, when accessing the variable inside the function when using bind, then we have to declare a new variable inside the function and assign it to the list variable using curselection method.