In this post we will learn about the text widget in Tkinter, Text is the best way to display a sentence or paragraph in the application. We will learn more about positioning and usage of Text below. Lets get started,
IN[1]
import tkinter as tk
from tkinter import ttk
try :
from ctypes import windll
windll.shcore.SetProcessDpiAwareness(1)
except :
pass
root = tk.Tk()
root.geometry("600x400")
root.resizable(False, False)
root.title("Text widgets in Tkinter")
text = tk.Text(root, height = 8)
root.mainloop()
OUT[1]
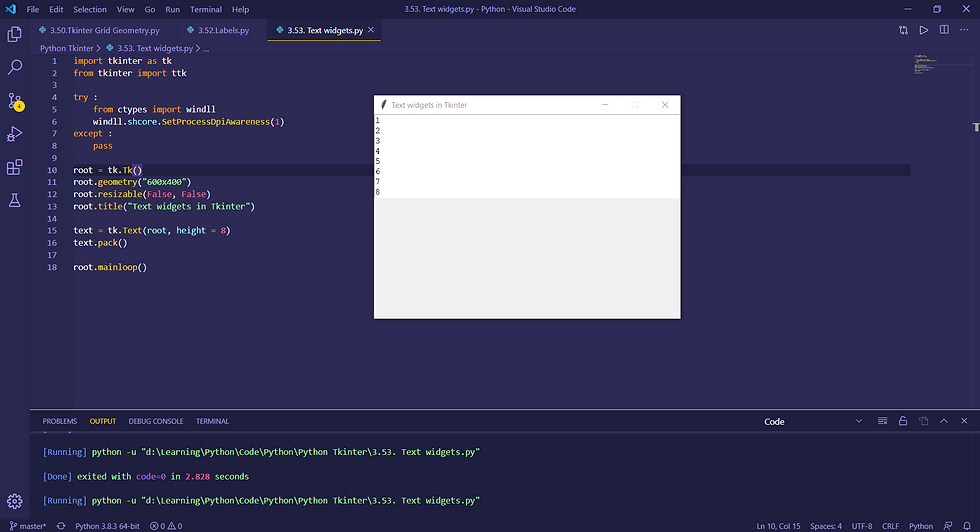
We declare the Text method with the height as an argument and then pack it to display it in our root window. If you notice, the opened window will have an editable text are inside the root window. As we have given the height as 8 the text will have 8 editable row in the root window.
IN[2]
import tkinter as tk
from tkinter import ttk
try :
from ctypes import windll
windll.shcore.SetProcessDpiAwareness(1)
except :
pass
root = tk.Tk()
root.geometry("600x400")
root.resizable(False, False)
root.title("Text widgets in Tkinter")
text = tk.Text(root, height = 8)
text.pack()
text.insert("1.0", "Prathap Dominincsavio")
#text["state"] = "disabled"
text_content = text.get("1.0", "end")
print(text_content)
root.mainloop()
OUT[2]

Instead of writing text yourself in the text area available in the opened window, we can also pass string along side the text. To do that we have to use insert method of Text and pass 2 arguments, a index to specify the row and column and a string the text which needs to be passed.
We can also get that text using Text.get method which also hold same no of 2 arguments as mentioned above, which can be later used as an input for evaluation or for printing data to user.
State of the text can also be specified, by default it is enabled for the text widgets created. If you wish to make that text space in-editable pass the argument as disabled.